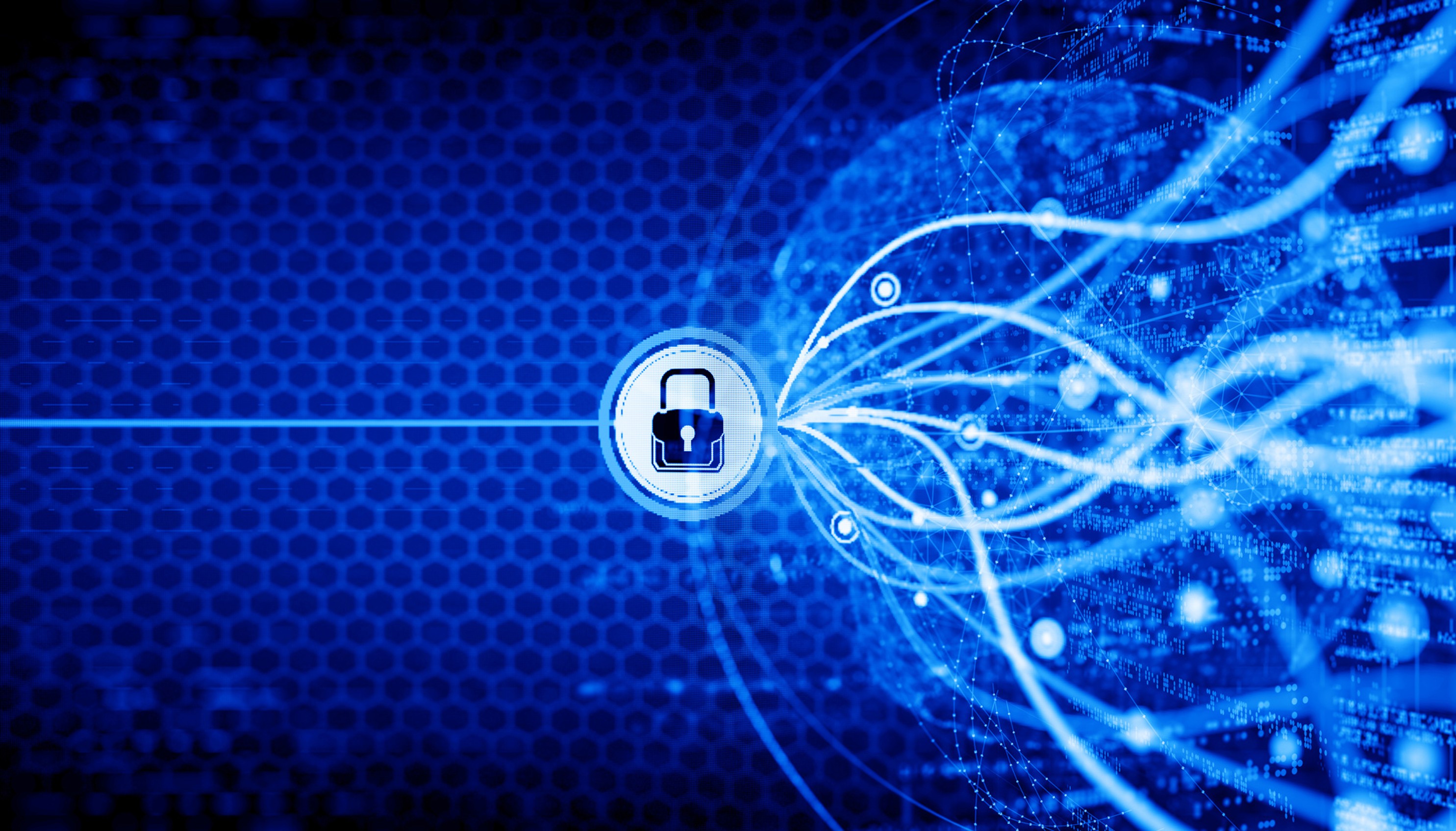
Security headers protect web applications from vulnerabilities like Cross-Site Scripting (XSS), clickjacking, and information leaks. This article explains key security headers, common issues, and how to configure them using HTML, Nginx, and Node.js.
1. Content-Security-Policy (CSP)
What is CSP?
The Content-Security-Policy (CSP) header controls which resources (scripts, styles, images) browsers are allowed to load, protecting your website from XSS and data injection attacks.
Problem: Unsafe or Missing CSP
- No
base-uri
Directive: - Missing
base-uri
allows attackers to inject<base>
tags, redirecting relative URLs to malicious domains. - Overly Permissive
script-src:
- If
script-src
permits unsafe sources, attackers can execute malicious scripts. - Use of
unsafe-inline
orunsafe-eval:
- Allows inline JavaScript or eval-based code execution, enabling XSS.
Solution
Set a strict CSP to limit resource loading and execution.
HTML Implementation:
Add a <meta> tag to your HTML <head> section:
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://cdnjs.cloudflare.com 'nonce-random123'; object-src 'none'; base-uri 'self';">
What It Does:
default-src
'self': Restricts default resource loading to the same domain.script-src
'self' https: //cdnjs.cloudflare.com 'nonce-random123': Allows scripts from your domain and trusted CDNs, using nonce for inline scripts.object-src
'none': Blocks plugins like Flash or Java.base-uri
'self': Prevents injectedtags from altering URLs.
Nginx Configuration:
add_header Content-Security-Policy "default-src 'self'; script-src 'self' https://cdnjs.cloudflare.com 'nonce-random123'; object-src 'none'; base-uri 'self';";
Node.js (Express):
app.use((req, res, next) => {
const nonce = crypto.randomBytes(16).toString('base64');
res.setHeader("Content-Security-Policy", `default-src 'self'; script-src 'self' https://cdnjs.cloudflare.com 'nonce-${nonce}'; object-src 'none'; base-uri 'self';`);
next();
});
2. X-Content-Type-Options
What is X-Content-Type-Options?
This header ensures browsers respect the declared Content-Type
of files, blocking MIME-type sniffing.
Problem: Missing X-Content-Type-Options
Without this header, browsers may treat non-executable files (e.g., images or text) as executable scripts, enabling XSS or phishing attacks.
Solution
Set the X-Content-Type-Options
header to nosniff
.
HTML Implementation:
Add a <meta> tag to your HTML <head> section:
<meta http-equiv="X-Content-Type-Options" content="nosniff">
Nginx Configuration:
add_header X-Content-Type-Options "nosniff";
Node.js (Express):
app.use((req, res, next) => {
res.setHeader("X-Content-Type-Options", "nosniff");
next();
});
3. Referrer-Policy
What is Referrer-Policy?
This header controls how much information about the referring page is shared with external sites. It helps protect sensitive URL data
from being leaked.
Problem: Missing Referrer-Policy
Without this header, browsers send complete URLs of referring pages to external sites, exposing sensitive data such as session tokens or unique identifiers.
Solution
Set the Referrer-Policy
header to no-referrer
.
HTML Implementation:
Add a <meta> tag to your HTML <head> section:
<meta name="referrer" content="no-referrer">
Nginx Configuration:
add_header Referrer-Policy "no-referrer";
Node.js (Express):
app.use((req, res, next) => {
res.setHeader("Referrer-Policy", "no-referrer");
next();
});
4. X-XSS-Protection
What is X-XSS-Protection?
The X-XSS-Protection
header activates the browser's built-in XSS protection filter, preventing malicious scripts from executing in reflected XSS attacks.
Problem: Missing X-XSS-Protection
Without this header, browsers may not block XSS attempts, leaving users vulnerable to attacks.
Solution:
Set the X-XSS-Protection
header to 1; mode=block
.
HTML Implementation:
Add a <meta> tag to your HTML <head> section:
Nginx Configuration:
add_header X-XSS-Protection "1; mode=block";
Node.js (Express):
app.use((req, res, next) => {
res.setHeader("X-XSS-Protection", "1; mode=block");
next();
});
Combining All Headers in HTML
Here’s how to implement all discussed security headers using <meta> tags in your HTML file:
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://cdnjs.cloudflare.com 'nonce-random123'; object-src 'none'; base-uri 'self';">
<meta http-equiv="X-Content-Type-Options" content="nosniff">
<meta name="referrer" content="no-referrer">
<meta http-equiv="X-XSS-Protection" content="1; mode=block">
Testing Your Security Headers
Here’s how to implement all discussed security headers using <meta> tags in your HTML file:
- SecurityHeaders.com: Checks your HTTP response headers.
- Browser Developer Tools:
- Open Developer Tools (F12).
- Go to the "Network" tab.
- Inspect the HTTP response headers for your website.
Conclusion
Security headers protect your website against XSS, clickjacking, and data leakage. Implementing these headers using HTML, Nginx, or Node.js ensures a secure browsing experience for your users. This guide provides actionable solutions for developers and non-developers, with copy-paste-ready examples to improve your website's security posture.